
A Brickstuff BrickPixel example
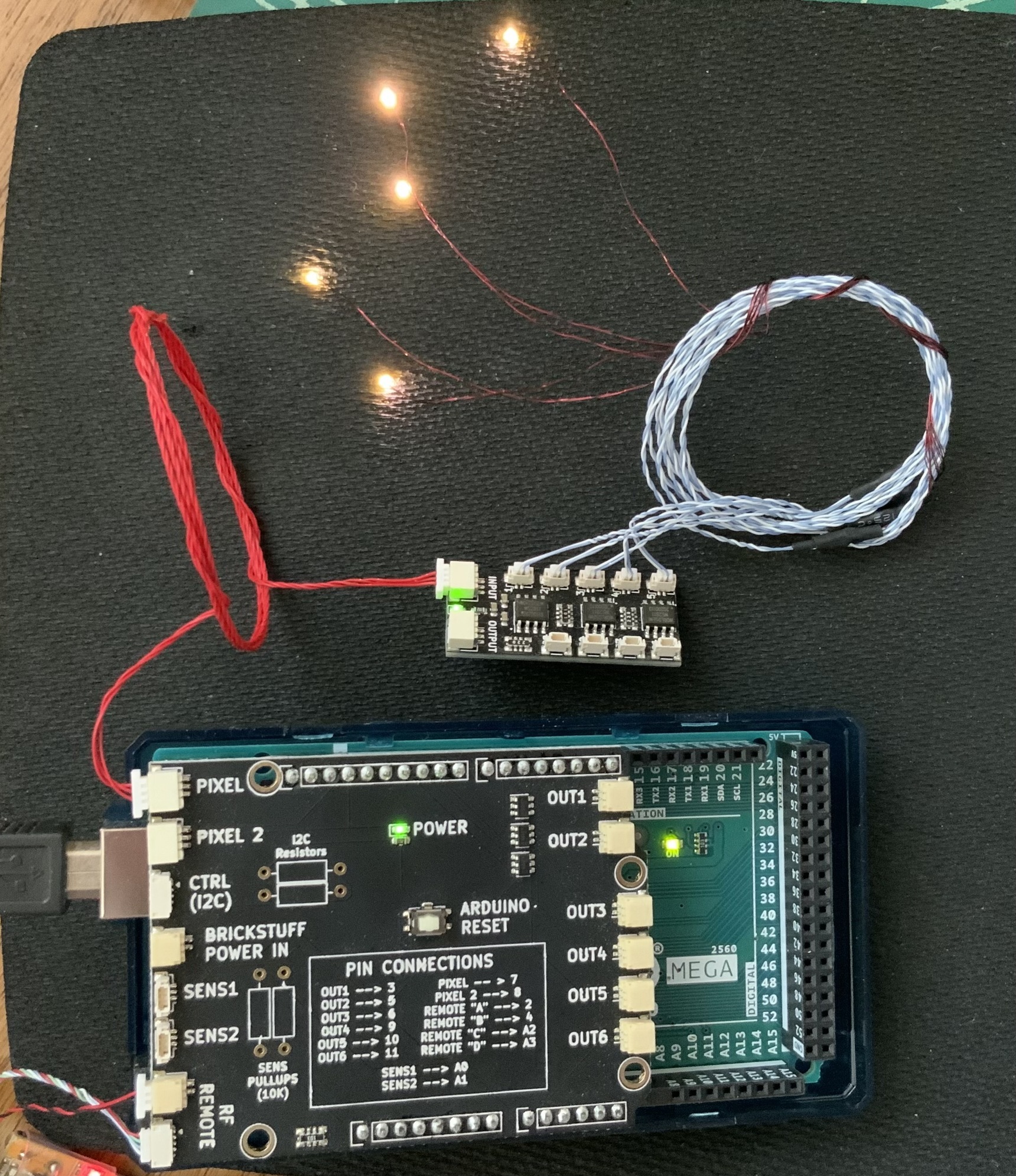
Above is the hardware setup for this BrickPixel example. The Brickstuff Arduino shield is connected to an Arduino Mega board. The Arduino Mega board is getting power through its USB port.
The shield's Pixel #1 port is connected to a Brickstuff BrickPixel 9-LED Light Control Board. Brickstuff custom 1mA warm white lights are plugged into the first five connectors on the board.
This is the heavily commented code running on the Arduino. Realize that I'm am not a programmer, so this program is neither efficient nor elegant...but it works and shows my current understanding of the hardware and software.
If this helps you out or you use any of it, let me know. Would love to see what other folks do with the BrickPixel system.
Thanks!
-wrtyler-
//////////////////////////// // Brickstuff Arduino Shield // // Sample code for adding RF remote control functionality // (c)2021, Bricktectonics, LLC // // THIS CODE IS PROVIDED AS-IS WITH NO WARRANTY OR GUARANTEE OF ANY KIND // // HISTORY // 05.24.2021 Version 1.0 // 05.27.2021 modified by wrtyler to show different light control techniques //////////////////////////// // OVERVIEW of modification // // This is a test program to drive six lights connected to a Brickstuff // BrickPixel 9-connector board. Five of the lights are Brickstuff custom // 3mA warm white LEDs and one light is a custom 1mA warm white LED. // The 3mA LEDs are used in Bricktectonic custom Mall lights and the // 1mA LED is used to light a food kiosk. // // The food kiosk light is programmed to toggle on and off as if someone // flipped a light switch in the building. // Four of the five Mall lighs smoothly fade up from black to maximum // brightness and then fade to black. // The fifth Mall light fades up from black, but flickers as it does so // as if the light is starting to go bad. It fades to black like // the other Mall lights. // // The program starts as soon as it is loaded into the Arduino. There is // no programmed trigger to start and stop the lighting effects. // #include <Adafruit_NeoPixel.h> // BrickPixel controls are on Pin 7 & 8 // Pin 7 = PIXEL 1, Pin 8 = PIXEL 2 #define PIN 7 // The AdaFruit software is designed to work with triplets of lights (R,G & B) // The Brickstuff hardware is designed to work with individual single color // lights. So, one Adafruit light is the same as three Brickstuff lights. // This is handled by the NUMPIXELS definition. // To calculate the NUMPIXELS, take the total number of Brickstuff lights that // are connected and divide by 3. If there is any remainder, increase // NUMPIXELS by 1. // In this example there are 6 Brickstuff lights attached. // 6/3 = 2 with no remainder. So, 2 for the quotient + 0 for the remainder. #define NUMPIXELS 2 // Declare a NeoPixel object. This is used to control the individual lights. Adafruit_NeoPixel pixels ( NUMPIXELS, PIN, NEO_RGB + NEO_KHZ800 ); // Argument 1 = Number of triplet groups of lights // Argument 2 = Arduino pin number of the BrickPixel socket being used // Argument 3 = Pixel type flags, add together as needed: // NEO_GRB Pixels are wired for GRB bitstream (most NeoPixel products) // NEO_RGB Pixels are wired for RGB bitstream (v1 FLORA pixels, not v2) // NEO_RGBW Pixels are wired for RGBW bitstream (NeoPixel RGBW products) // NEO_KHZ800 800 KHz bitstream (most NeoPixel products w/ WS2812 LEDs) // NEO_KHZ800 400 KHz (classic 'v1' (not v2) FLORA pixels, WS2811 drivers) void setup() { // Initialize the NeoPixel object (this is REQUIRED) pixels.begin(); // Set up the random number generator used for light flickering. randomSeed( random( 10000 ) ); } void loop() { // Clear out any prior BrickPixel settings pixels.clear(); // In this example, the brightness of the Brickstuff lights is looped from // 0 (off) to 255 (max brightness) in increments of 4 (to make it faster) for( int brightness=0; brightness<=255; brightness+=4 ) { // For each group of light triplets, set the brightness. for( int i=0; i<NUMPIXELS; i++ ) { // The first 3 lights are Mall lights and they smoothly fade from black // to full brightness. if( i < NUMPIXELS-1 ) { pixels.setPixelColor( i, pixels.Color( fadeOn( brightness ), fadeOn( brightness ), fadeOn( brightness ) ) ); } // The next 3 lights: the first on flickers as it fades from black to // full brightness, the second fades smoothly from black to full // brightness, the third toggles on immediately. else { pixels.setPixelColor( i, pixels.Color( flickerOn( brightness ), fadeOn( brightness ), toggleOn() ) ); } // Send the brightness settings to the BrickPixel controller. pixels.show(); } // Add a delay so the flickering doesn't happen so fast that you can't // see it delay( 60 ); } // Keep the lights at full brightness for two seconds delay( 2000 ); // Once the lights are at full intensity, smoothly reduce the brightness // until they are all completely dark for( int brightness=255; brightness>=0; brightness-- ) { for( int i=0; i<NUMPIXELS; i++ ) { // Smoothly fade the five Mall lights to black and toggle the kiosk // off immediately if( i < NUMPIXELS-1 ) { pixels.setPixelColor( i, pixels.Color( fadeOff( brightness ), fadeOff( brightness ), fadeOff( brightness ) ) ); } else { pixels.setPixelColor( i, pixels.Color( fadeOff( brightness ), fadeOff( brightness ), toggleOff() ) ); } // Send the brightness settings to the BrickPixel controller. pixels.show(); } // Add a slight delay so the fade to black doesn't occur too quickly delay( 2 ); } // Keep the lights off for one second delay( 1000 ); } /* * Functions for specific light controls */ // Set the brightness to max brightness uint8_t toggleOn() { return 255; } uint8_t fadeOn( uint8_t brightness ) { return( brightness ); } // To create flickering, multiply the brightness by a random number between // 0 and 1. When the value is close to max brightness, set the light to // max brightness. uint8_t flickerOn( uint8_t brightness ) { // Is the brightness close to max brightness? If yes, return max brightness if( brightness > 251 ) { return 255; } // If no, return a random brightness else { return( brightness * ( random(100) / 100.0 ) ); } } // Set the brightness to 0 uint8_t toggleOff() { return 0; } uint8_t fadeOff( uint8_t brightness ) { return( brightness ); }